What are Micro Interactions
Table of contents
- What Are Micro Interactions?
- Why Micro Interactions Are Essential
- Micro Interactions vs. Animations
- Examples of Micro Interactions
- Micro Interactions in Social Theory
- Designing Effective Micro Interactions
- Tools for Creating Micro Interactions
- Micro Interactions in Marketing
- Microcultures in Marketing
- Conclusion
Have you ever heard someone recount a hiring experience that went exceptionally well? Chances are, they mentioned a brief moment of approval from the hirer’s face or a small gesture that positively caught the boss’s attention. Similarly, if you’ve visited a professional fine dining kitchen, you’ve likely noticed subtle details that made your experience truly unforgettable.
Micro-interactions are small design details, like a heart animation when you like a post or a loading spinner, that give quick feedback and make using apps or websites feel smooth and engaging.
Today we’ll focus on digital micro-interactions designed to capture your users’ or clients’ attention. With simple UI or UX tools, you can positively influence users’ perceptions. By studying their routines and behaviors, you can implement micro interactions that are either functional or visually appealing.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Micro-Interactions</title>
<style>
/* General Styles */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 1rem;
display: flex;
flex-direction: column;
align-items: center; /* Centers horizontally */
text-align: center;
gap: 1rem;
}
h3 {
font-size: 1rem;
margin: 0.5rem 0;
}
/* Button Interaction */
.btn {
background: #4CAF50;
color: white;
border: none;
padding: 0.5rem 1rem;
border-radius: 0.25rem;
cursor: pointer;
transition: background 0.3s, transform 0.2s;
}
.btn:hover {
background: #388E3C;
}
.btn:active {
transform: scale(0.95);
}
/* Like Button */
.like-btn {
font-size: 1.5rem;
cursor: pointer;
transition: transform 0.2s, color 0.3s;
}
.like-btn.liked {
color: red;
transform: scale(1.2);
}
/* Form Validation */
.form-container {
display: flex;
flex-direction: column;
align-items: center; /* Centers horizontally */
gap: 0.5rem;
}
.input {
width: 80%;
max-width: 20rem;
padding: 0.5rem;
border: 1px solid #ccc;
border-radius: 0.25rem;
text-align: center;
}
.input.invalid {
border-color: red;
animation: shake 0.3s ease-in-out;
}
@keyframes shake {
0%, 100% { transform: translateX(0); }
25% { transform: translateX(-5%); }
75% { transform: translateX(5%); }
}
.submit-btn {
background: #0078D7;
color: white;
border: none;
padding: 0.5rem 1rem;
border-radius: 0.25rem;
cursor: pointer;
transition: background 0.3s;
}
.submit-btn:hover {
background: #005BB5;
}
</style>
</head>
<body>
<h3>1. Button Interaction</h3>
<button class="btn">Click Me!</button>
<h3>2. Like Interaction</h3>
<span id="likeBtn" class="like-btn">❤️</span>
<h3>3. Form Validation</h3>
<div class="form-container">
<input type="text" id="username" class="input" placeholder="Enter Username">
<input type="password" id="password" class="input" placeholder="Enter Password">
<button class="submit-btn" onclick="validateForm()">Submit</button>
</div>
<script>
// Like Button Interaction
const likeBtn = document.getElementById('likeBtn');
likeBtn.addEventListener('click', () => {
likeBtn.classList.toggle('liked');
});
// Form Validation Interaction
function validateForm() {
const username = document.getElementById('username');
const password = document.getElementById('password');
if (username.value.trim() === '') {
username.classList.add('invalid');
setTimeout(() => username.classList.remove('invalid'), 500);
}
if (password.value.trim().length < 6) {
password.classList.add('invalid');
setTimeout(() => password.classList.remove('invalid'), 500);
}
}
</script>
</body>
</html>
Over time, these UI micro-interactions become so ingrained in daily routines that we barely notice using them. For example, have you just encountered one without realizing it? Let’s say you were searching for information on micro interactions in your browser. As you began typing your keywords, suggestions appeared in the search bar to guide you. That visual cue is also an example of a micro-interaction.
As shown, these straightforward interactions can significantly enhance a user’s experience on a website. But how can you create your own or even recognize them to start? In today’s article, we’ll explore micro-interactions from various perspectives. Get ready to shift your perception—you’ll soon find yourself actively seeking out micro-interactions once you learn more about them!
What Are Micro Interactions?
Micro-interactions are critical small moments in digital interfaces that significantly impact user engagement, ease of use, feedback, and functionality. They often take the form of visual or animated elements on apps or websites. For a micro-interaction to function effectively for the user, certain components need to be prioritized. These components include:
- Trigger: Any action that starts the interaction, such as a click, typing on a keyboard, a voice command, or a hover, can serve as a trigger. The choice of trigger depends on the specific user scenario you are designing for.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Micro-Interaction Trigger Example</title>
<style>
.button {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
transition: transform 0.1s ease-in-out;
}
.button:active {
transform: scale(0.95);
}
</style>
</head>
<body>
<h3>Trigger Example</h3>
<button class="button">Click Me!</button>
</body>
</html>
- Rules: The steps that follow the trigger define the rules. These are the logical sequences that create the reactions, such as button clicks leading to animations, opening screens, or loading indicators.
- Feedback: Responses that inform the user about what is happening are referred to as feedback. Examples include loading indicators, sign-in messages, alert animations, or visuals. For instance, if a password is entered incorrectly, a red exclamation mark may appear next to the password field.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Password Feedback Example</title>
<style>
.input-container {
margin: 20px 0;
}
.password {
border: 2px solid #ccc;
padding: 8px;
border-radius: 4px;
}
.password.invalid {
border-color: red;
animation: shake 0.3s ease-in-out;
}
@keyframes shake {
0%, 100% { transform: translateX(0); }
25% { transform: translateX(-5px); }
75% { transform: translateX(5px); }
}
</style>
</head>
<body>
<h3>Password Feedback</h3>
<div class="input-container">
<input type="password" id="password" class="password" placeholder="Enter Password">
<button onclick="validatePassword()">Submit</button>
</div>
<script>
function validatePassword() {
const passwordInput = document.getElementById('password');
if (passwordInput.value.length < 6) {
passwordInput.classList.add('invalid');
setTimeout(() => passwordInput.classList.remove('invalid'), 500);
}
}
</script>
</body>
</html>
- Loops and Modes: These represent the repetitive or continuous behaviors within the system. They govern how micro-interactions function over time and determine their duration or recurrence.
Why Micro Interactions Are Essential
So far, we have explored what is micro-interactionism. As observed, the components establish a logical process that ensures these micro-interactions are both functional and capable of providing visual and audible feedback. With these elements, you can craft engaging task-based interactions.
These interaction responses can help enhance user retention while showcasing your brand identity. Incorporating your mascot, slogan, logo, or other brand visual elements into the design of your micro-interactions can make them more personalized. Well-designed micro-interactions also highlight your attention to detail.
Micro Interactions vs. Animations
If you are new to micro-interactions, one of the first questions you might have is what is the difference between micro-interactions and animation. While both contribute to making apps and websites more engaging and enjoyable, they have distinct characteristics.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Micro-Interactions vs Animations</title>
<style>
/* General Styles */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 1rem;
display: flex;
flex-direction: column;
align-items: center;
text-align: center;
gap: 2rem;
}
h2 {
margin-bottom: 0.5rem;
}
/* Micro-Interaction Button */
.micro-btn {
background: #4CAF50;
color: white;
border: none;
padding: 0.7rem 1.5rem;
border-radius: 5px;
cursor: pointer;
transition: background 0.3s, transform 0.1s;
position: relative;
}
.micro-btn:active {
transform: scale(0.95);
}
.micro-btn .spinner {
display: none;
border: 2px solid white;
border-radius: 50%;
border-top: 2px solid transparent;
width: 15px;
height: 15px;
position: absolute;
right: -25px;
top: 50%;
transform: translateY(-50%);
animation: spin 1s linear infinite;
}
@keyframes spin {
0% { transform: translateY(-50%) rotate(0deg); }
100% { transform: translateY(-50%) rotate(360deg); }
}
.micro-btn.loading .spinner {
display: inline-block;
}
.micro-btn.done::after {
content: '✔️';
position: absolute;
right: -25px;
top: 50%;
transform: translateY(-50%);
}
/* Animation Transition */
.animation-container {
width: 100%;
height: 200px;
display: flex;
align-items: center;
justify-content: center;
overflow: hidden;
background: #f0f0f0;
border: 1px solid #ddd;
position: relative;
}
.thank-you {
width: 100%;
height: 100%;
background: #FFD700;
display: flex;
align-items: center;
justify-content: center;
font-size: 1.5rem;
position: absolute;
top: 0;
left: 100%;
transition: left 0.7s ease-in-out;
}
.animation-container.active .thank-you {
left: 0;
}
.confetti {
position: absolute;
width: 5px;
height: 5px;
background: red;
top: -10px;
animation: fall 2s ease-out infinite;
}
@keyframes fall {
0% { transform: translateY(0); opacity: 1; }
100% { transform: translateY(200px); opacity: 0; }
}
</style>
</head>
<body>
<h1>Micro-Interactions vs Animations</h1>
<!-- Micro-Interaction Example -->
<h2>1. Micro-Interaction Example</h2>
<button id="microBtn" class="micro-btn">
Download
<div class="spinner"></div>
</button>
<!-- Animation Example -->
<h2>2. Animation Example</h2>
<div class="animation-container" id="animationContainer">
<div class="thank-you">🎉 Thank You for Downloading! 🎉</div>
</div>
<script>
// Micro-Interaction Logic
const microBtn = document.getElementById('microBtn');
const animationContainer = document.getElementById('animationContainer');
let isDownloadTriggered = false; // Prevent multiple triggers
microBtn.addEventListener('click', () => {
if (isDownloadTriggered) return; // Prevent multiple triggers
isDownloadTriggered = true;
// Micro-Interaction Feedback
microBtn.classList.add('loading');
microBtn.textContent = 'Downloading...';
setTimeout(() => {
microBtn.classList.remove('loading');
microBtn.classList.add('done');
microBtn.textContent = 'Completed';
// Trigger Animation After Download Completes
animationContainer.classList.add('active');
launchConfetti();
}, 1000); // Reduced download time to 1 second
});
// Confetti Animation Logic
function launchConfetti() {
for (let i = 0; i < 50; i++) {
const confetti = document.createElement('div');
confetti.classList.add('confetti');
confetti.style.left = Math.random() * 100 + 'vw';
confetti.style.animationDelay = Math.random() * 2 + 's';
animationContainer.appendChild(confetti);
}
}
</script>
</body>
</html>
Micro-Interactions: These are task-based responses designed to guide users through their online journey. They often include visual or audible feedback that serves a functional purpose for the user. Despite their small size, they have a significant impact on the overall experience of the digital product. For example, think of a like button that animates a heart when clicked.
Animations: These are more focused on creating visual engagement and serving as a storytelling tool for apps and websites. Their aesthetic nature makes them ideal for use in transition effects. For instance, remember the excitement of discovering PowerPoint transitions in middle school—animations are the professional equivalent of that. A full-screen transition between app pages is a typical example.
As we’ve explained, micro-interactions are primarily functionality-driven, whereas animations are more focused on aesthetics. A simple example can illustrate this distinction:
If a user clicks on a DOWNLOAD button and it changes size upon being clicked, this is a micro-interaction. However, if the page transitions to a payment page with a sliding transformation effect, this is considered an animation.
Examples of Micro Interactions
We encounter micro-interactions frequently, often without realizing it. Over the years, as user interaction and experience design have evolved, the use of micro-interactions has become more widespread. Even something as simple as scrolling through a page can reveal glimpses of micro-interactions—give it a try! It seems this blog has become a micro-interacting experience itself :)
UI Applications
- Button hover effects are among the most common examples, especially with call-to-action buttons. If you’ve seen task-driven buttons like DOWNLOAD, BUY, or CHECK OUT, you might notice slight design changes when you click or hover over them. These subtle changes help grab attention, even from users who are just browsing the site.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CTA Hover Interaction</title>
<style>
.cta-button {
background: #0078D7;
color: white;
padding: 12px 24px;
border: none;
border-radius: 4px;
cursor: pointer;
transition: background 0.3s, transform 0.2s;
}
.cta-button:hover {
background: #005BB5;
transform: translateY(-3px);
}
</style>
</head>
<body>
<h3>CTA Button</h3>
<button class="cta-button">Learn More</button>
</body>
</html>
- Scroll progress indicators are commonly used in blogs, articles, or stories on websites. They show readers how much content remains, helping them gauge the reading time or progress.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Scroll Progress Indicator</title>
<style>
.progress-container {
width: 100%;
height: 8px;
background: #ccc;
position: fixed;
top: 0;
left: 0;
z-index: 1000;
}
.progress-bar {
height: 100%;
background: #4caf50;
width: 0;
}
.content {
height: 2000px;
}
</style>
</head>
<body>
<div class="progress-container">
<div class="progress-bar" id="progressBar"></div>
</div>
<div class="content"></div>
<script>
window.addEventListener('scroll', () => {
const progressBar = document.getElementById('progressBar');
const scrollPosition = document.documentElement.scrollTop;
const height = document.documentElement.scrollHeight - document.documentElement.clientHeight;
const scrolled = (scrollPosition / height) * 100;
progressBar.style.width = scrolled + '%';
});
</script>
</body>
</html>
- Swipe-to-delete gestures are primarily seen on phones or tablets since they rely on finger swipes. These are commonly found in email or direct messaging applications.
E-commerce Micro Interaction Examples:
- Real-time cart updates allow users to view their current cart and shopping status. This helps users track how much they’ve added and calculate their total. Including details like discounts can even encourage additional purchases.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Add to Wishlist Animation</title>
<style>
/* General Styles */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 1rem;
display: flex;
flex-direction: column;
align-items: center;
text-align: center;
gap: 2rem;
}
h2 {
font-size: 1.2rem;
margin-bottom: 0.5rem;
}
/* Add to Wishlist Button */
.wishlist-btn {
background: #ff4757;
color: white;
border: none;
padding: 0.7rem 1.5rem;
border-radius: 5px;
cursor: pointer;
transition: background 0.3s, transform 0.2s;
position: relative;
}
.wishlist-btn.added {
background: #2ed573;
}
.wishlist-btn .heart {
position: absolute;
font-size: 1.2rem;
top: -10px;
right: -10px;
opacity: 0;
transform: scale(0.5);
animation: pop 0.5s ease-out forwards;
}
@keyframes pop {
0% { transform: scale(0.5); opacity: 0; }
50% { transform: scale(1.2); opacity: 1; }
100% { transform: scale(1); opacity: 0; }
}
</style>
</head>
<body>
<h2>🛒 Add to Wishlist Animation</h2>
<button id="wishlistBtn" class="wishlist-btn">
Add to Wishlist
<span class="heart">❤️</span>
</button>
<script>
const wishlistBtn = document.getElementById('wishlistBtn');
wishlistBtn.addEventListener('click', () => {
wishlistBtn.classList.toggle('added');
wishlistBtn.innerText = wishlistBtn.classList.contains('added')
? 'Added to Wishlist'
: 'Add to Wishlist';
const heart = wishlistBtn.querySelector('.heart');
heart.style.animation = 'none';
setTimeout(() => heart.style.animation = 'pop 0.5s ease-out forwards', 0);
});
</script>
</body>
</html>
- “Add to wishlist” animations work similarly to real-time cart updates, enabling customers to manage their lists and use them as checklists.
Everyday Examples
- Typing indicators in messaging apps are excellent examples, mimicking real-life communication. They make interactions smoother and evoke emotions depending on who is typing—whether it’s a loved one or your boss!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Typing Indicator Interaction</title>
<style>
/* General Styles */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 1rem;
display: flex;
flex-direction: column;
align-items: center;
text-align: center;
gap: 1.5rem;
}
h2 {
font-size: 1.2rem;
margin-bottom: 0.5rem;
}
/* Chat Container */
.chat-container {
display: flex;
flex-direction: column;
align-items: center;
gap: 0.5rem;
width: 80%;
max-width: 400px;
}
.chat-input {
width: 100%;
padding: 0.7rem;
border: 1px solid #ccc;
border-radius: 5px;
text-align: center;
font-size: 1rem;
}
.typing-indicator {
font-size: 0.9rem;
color: #555;
visibility: hidden;
display: flex;
align-items: center;
gap: 0.3rem;
}
.typing-indicator.visible {
visibility: visible;
}
.dot {
width: 6px;
height: 6px;
background: #555;
border-radius: 50%;
animation: blink 1.5s infinite;
}
.dot:nth-child(2) {
animation-delay: 0.2s;
}
.dot:nth-child(3) {
animation-delay: 0.4s;
}
@keyframes blink {
0%, 100% { opacity: 0.2; }
50% { opacity: 1; }
}
</style>
</head>
<body>
<h2>💬 Typing Indicator Interaction</h2>
<div class="chat-container">
<input
type="text"
id="chatInput"
class="chat-input"
placeholder="Start typing something..."
/>
<div id="typingIndicator" class="typing-indicator">
<span>Typing</span>
<div class="dot"></div>
<div class="dot"></div>
<div class="dot"></div>
</div>
</div>
<script>
const chatInput = document.getElementById('chatInput');
const typingIndicator = document.getElementById('typingIndicator');
let typingTimeout;
chatInput.addEventListener('input', () => {
typingIndicator.classList.add('visible');
clearTimeout(typingTimeout);
typingTimeout = setTimeout(() => {
typingIndicator.classList.remove('visible');
}, 1000); // Hides indicator after 1 second of inactivity
});
</script>
</body>
</html>
- Notifications and alerts are other daily examples, especially on phones. These can be system notifications or alerts directly from websites.
Micro Interactions in Social Theory
Symbolic Interactionism:
Since the field of user interaction intersects with sociology in developing tools, micro-interactions are also connected to it. You might wonder which sociological theory focuses on micro-level interactions.
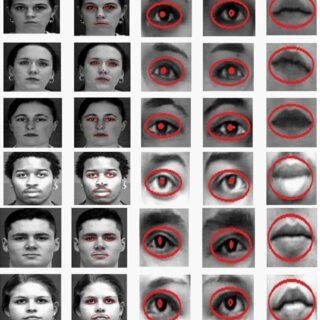
These small-scale interactions derive their meaning by reflecting real-life interactions. In face-to-face encounters that occur in everyday life, people naturally express responses to small, momentary exchanges. Micro-interactions examples allow users to replicate these social encounters in texting, video calls, or direct messaging. Therefore, any sociological perspective that studies how individuals create meaning through small-scale interactions can serve as an overarching framework for these concepts.
Designing Effective Micro Interactions
Start with the User: Since UI micro-interactions are function-driven, designing them based on users’ needs is crucial. By analyzing users’ behaviors when interacting with your digital products, you can create designs tailored to their requirements.
Keep It Purposeful: It’s essential to establish a clear goal for each micro-interaction. Adding micro-animations and interactions indiscriminately or for any trigger can become overwhelming and detract from the user experience.
Focus on Feedback: Providing feedback during processes like purchasing, reading, or through audible or visual alerts is vital for effective micro-interactions. Clear and immediate feedback enhances usability and ensures a smoother experience.
Maintain Consistency: Consistency and harmony in visual design are key. Once you’ve defined your goals and determined where to implement micro-interactions, ensure they align with each other and the overall design language of your product.
Don't Overwhelm: Overusing micro-interactions and animations can frustrate users and risk making your site appear unprofessional—resembling a flash gaming website for children. To prevent information overload, carefully limit the amount of micro-interactions you include.
Tools for Creating Micro Interactions
- The most common tools for prototyping micro-interaction examples are Figma and Adobe XD. Their user-friendly interfaces allow you to quickly adapt, even if you’re not very familiar with the software, making it easy to create your designs.
- When it comes to animations, it's best to rely on industry favorites. Principle and After Effects are ideal for advanced animations that can be adapted to any user interface, app, or website. Both programs offer great potential for enhancing your skills in creating visual animations.
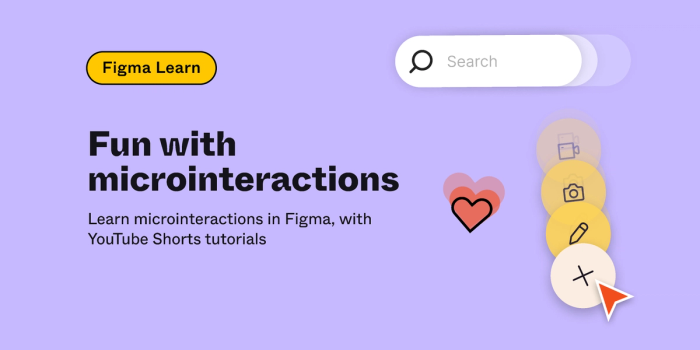
Micro Interactions in Marketing
Do you remember the time when Microsoft Office documents had an assistant on the screen? It would provide feedback and small tips on how to navigate the document. For many users, the assistant’s eyes following the mouse felt almost magical and motivated them to work better. Over time, designs have become richer, but our appreciation for simple interactions and animations remains unchanged.
Applications in Email Marketing
Enhancing Engagement: In email marketing, the main goal is often to guide users toward taking an action—whether it's completing a purchase, downloading an e-book, or visiting a website. To boost engagement, you can design hover effects for your call-to-action buttons to encourage clicks. These simple, surprising interactions can motivate your recipients to click.

Personalizing Experience: Special offers and the fear of missing out (FOMO) are common selling tactics. You can apply these in your online store by adding a countdown for promotions or animated progress bars for limited-time offers within your email.
Incorporating Micro-Interactions in Email Templates
Interactive Buttons: Visual elements capture attention in a simple yet effective way. To improve engagement metrics in your emails, incorporate micro-interactions in your CTAs. For example, you can add size or color change animations to buttons when users hover over them.
Dynamic Elements: Email templates don’t have to be static or just text-based. You can include animations like simple icons or spinning loaders for processing forms. These interactive elements enhance the user experience.
Celebratory Animations: Rewarding interactions or animations help users feel a sense of familiarity and joy towards your business. For example, you can use confetti effects or celebratory animations for completed purchases, special offers, or anniversary campaigns.
Microcultures in Marketing
Microcultures refer to segments of subgroups with distinct preferences and behaviors in marketing. For example, older generations who may struggle to understand the small details on websites will require larger buttons and more noticeable animations to stay engaged.
Application: Leveraging user data is crucial in marketing as it can provide detailed solutions to your business's specific challenges. You can tailor micro-interactions to resonate with particular audience segments. By analyzing user behaviors and purchasing habits, you can create personalized micro-interactions.
For instance, if your primary user persona is the younger generation, you might focus more on gamification. With game-like transformations, animations, and interactions, you can create a sense of familiarity. These simple interactions will help make your brand more memorable.
Conclusion
We’ve seen that for designers and marketers, micro-interactions play a significant role in shaping the user experience on your website. Regardless of how small the design of your micro-interaction or animation may be, as long as it is visible and contributes functionally or aesthetically to your design, its impact will be profound.
These micro-interactions also serve as subtle yet powerful tools for conveying your brand’s identity and enhancing its personality, ultimately creating a deeper connection with users. By maintaining harmony in design elements, you can foster a sense of familiarity across your website, incorporating consistent colors and animations in hover buttons or alerts.
We hope this article has helped you envision memorable micro-interactions that align seamlessly with your website and brand. Happy emailing!